Sharing your SageMaker model
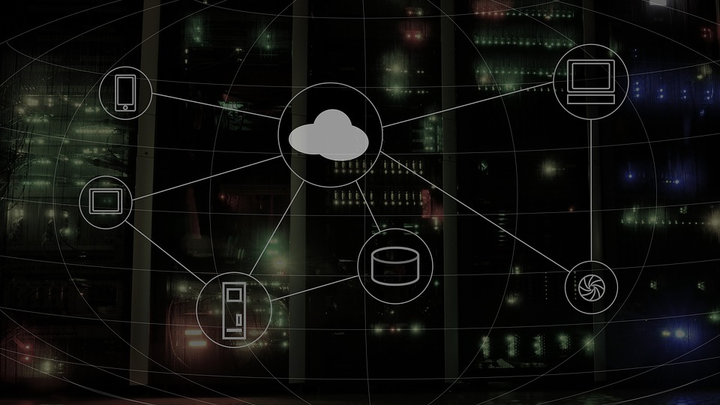
Introduction
AWS recently announced SageMaker, which helps you do everything from building models from scratch to deploying and scaling those models for use in production. This article will assume you already have a model running in SageMaker and want to grant access to another AWS account to make predictions with your model.
Grant permissions to the child account from the parent account
At a high level, you need to create a role that has access to invoke the endpoint, and then give the child account access to this role. For this, you’ll need the 12-digit account id of the child account. Then, follow these steps:
- Login to parent account Console and navigate to the IAM service
- Create new policy called “SageMakerExternalInvokeEndpoint”. Paste in the following policy. Note the data in brackets you’ll need to input.
{
"Version": "2012-10-17",
"Statement": [
{
"Action": ["sagemaker:InvokeEndpoint"],
"Effect": "Allow",
"Resource": "arn:aws:sagemaker:*:<parent_account_id>:endpoint/<endpoint_name>"
}
]
}
- Click “Roles” and create “SageMakerExternal” Role
- Choose “Another AWS Account” and paste child account_id
- Attach the new “SageMakerExternalInvokeEndpoint” policy (click “Policy type” arrow , “Customer Managed”, then select “SageMakerExternalInvokeEndpoint”)
- Click on the new role and copy the role arn, which will be used in later steps.
Grant “Assume Role” Policy to Child Account
This section will grant the ability to assume the role just created by the child account. You’ll need the role arn from the last step above.
- Login to Console -> IAM using child account
- If you don’t have a Group, create one. I called mine “SagemakerTester”. If you have existing users, you can add them to the group.
- Click “Permissions” tab, expand the “Inline Policies” arrow, and then Create Group Policy. Add this policy:
{
"Version": "2012–10–17",
"Statement": {
"Effect": "Allow",
"Action": "sts:AssumeRole",
"Resource": "<SageMakerExternal role arn copied above>"
}
}
- Use the Assumed Role in Boto3
- Now that the permissions are configured, you’ll need to be able to assume this role whenever you’re trying to hit the endpoint. If you’re using Boto3, the code will look something like this:
# Get temporary assumed role credentials
client = boto3.client('sts') #assumes you’ve configured boto with normal child account credentials
response = client.assume_role(
RoleArn="<SageMakerExternal role arn copied above>",
RoleSessionName="testSessionName", # this is arbitrary
ExternalId=‘<parent account_id>’
)
# Create boto client using temp credentials
client = boto3.client(
'sagemaker-runtime',
aws_access_key_id=response["Credentials"]["AccessKeyId"],
aws_secret_access_key=response["Credentials"]["SecretAccessKey"],
aws_session_token=response["Credentials"]["SessionToken"],
)
# Invoke endpoint
client.invoke_endpoint(
EndpointName=<endpoint_name>,
Body=<data>
)